Trilib插件为Unity3D的付费插件,可实现Unity程序运行状态下,从程序外部动态加载数模。支持FBX, OBJ, GLTF2, STL, PLY, 3MF以及ZIP等文件。
1.安装&编译
- 下载“TriLib - Model loader package.unitypackage”插件包,并使Unity3D导入插件;
- 编译运行时报错:TriLibConfigurePlugin.cs报错:没有”BuildTarget.Lumis”值,将其改为”BuildTarget.StandaloneWindows”,即可编译通过;
运行TriLib自带的AssetLoader场景,效果如下:
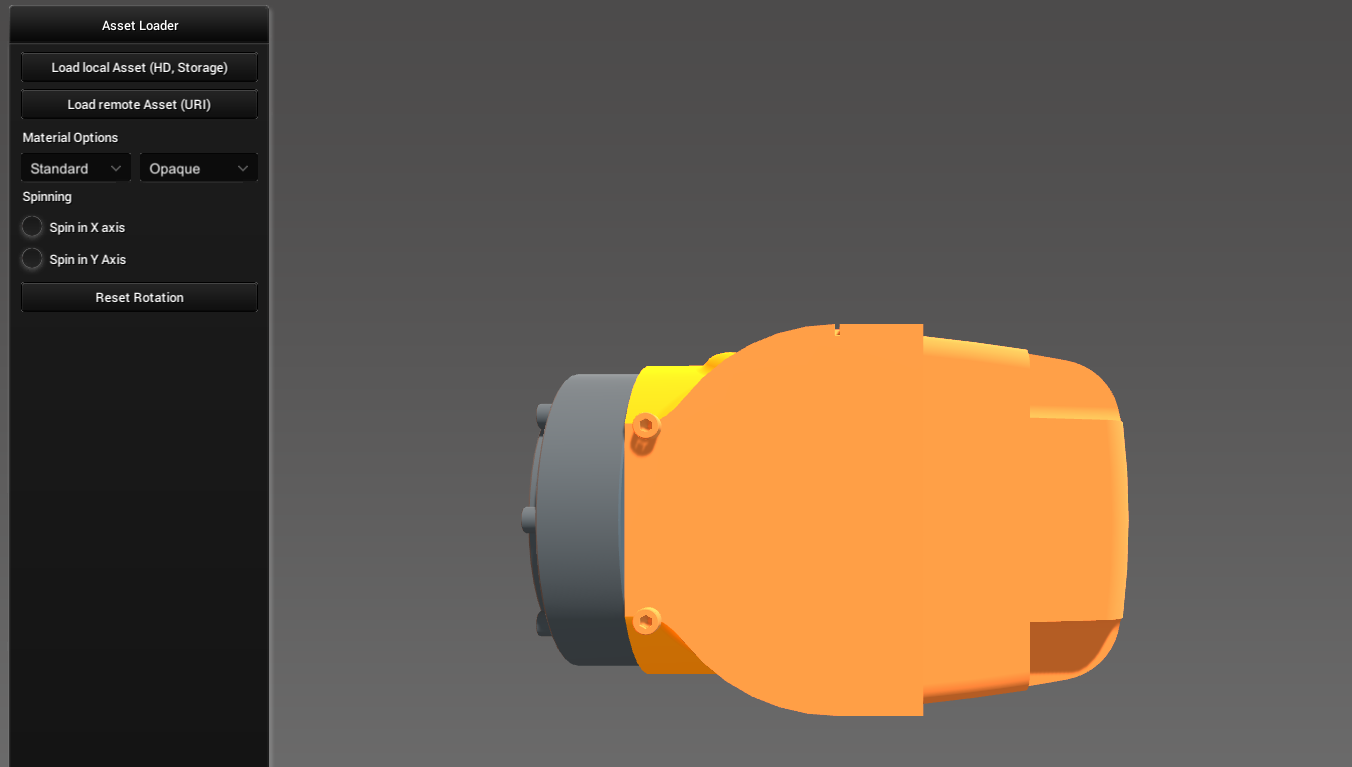
2.源码分析
2.1 Sample.cs进行修改
使用assetLoader类之间进行动态加载
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| public void LoadFBXClick() { string fbxPath = @"C:\Users\Administrator\Documents\ABB\J4.FBX"; using (var assetLoader = new AssetLoader()) { try { var assetLoaderOptions = AssetLoaderOptions.CreateInstance(); assetLoaderOptions.RotationAngles = new Vector3(90f, 180f, 0f); assetLoaderOptions.AutoPlayAnimations = true; assetLoaderOptions.UseOriginalPositionRotationAndScale = true; var loadedGameObject = assetLoader.LoadFromFile(fbxPath, assetLoaderOptions); loadedGameObject.transform.position = new Vector3(128f, 0f, 0f); } catch (Exception e) { Debug.LogError(e.ToString()); } } }
|
通过查看AssetLoaderWindow.cs
,发现主要实现AssetLoader
类调用
2.2 AssetLoaderWindow.cs中LoadInternal
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68
|
private void LoadInternal(string filename, byte[] fileBytes = null, int browserFilesCount = -1) { PreLoadSetup(); var assetLoaderOptions = GetAssetLoaderOptions(); if (!Async) { using (var assetLoader = new AssetLoader()) { assetLoader.OnMetadataProcessed AssetLoader_OnMetadataProcessed; try { if (fileBytes != null && fileBytes.Length > 0) { _rootGameObject = assetLoader.LoadFromMemoryWithTextures(fileBytes, FileUtils.GetFileExtension(filename), assetLoaderOptions, _rootGameObject); }else if (!string.IsNullOrEmpty(filename)) { _rootGameObject = assetLoader.LoadFromFileWithTextures(filename, assetLoaderOptions); }else { throw new Exception("File not selected"); } CheckForValidModel(assetLoader); }catch (Exception exception) { HandleException(exception); } } FullPostLoadSetup(); }else { using (var assetLoader = new AssetLoaderAsync()) { assetLoader.OnMetadataProcessed += AssetLoader_OnMetadataProcessed; try{
if (fileBytes != null && fileBytes.Length > 0) { assetLoader.LoadFromMemoryWithTextures(fileBytes, FileUtils.GetFileExtension(filename), assetLoaderOptions, null, delegate (GameObject loadedGameObject) { CheckForValidModel(assetLoader); _rootGameObject = loadedGameObject; FullPostLoadSetup(); }); }else if (!string.IsNullOrEmpty(filename)) { assetLoader.LoadFromFileWithTextures(filename, assetLoaderOptions, null, delegate (GameObject loadedGameObject) { CheckForValidModel(assetLoader); _rootGameObject = loadedGameObject; FullPostLoadSetup(); }); }else{ throw new Exception("File not selected"); } }catch (Exception exception) { HandleException(exception); } } } }
|
2.3 从文件夹一次性加载多个文件
1 2 3 4
| public void LoadFromBrowserFiles(int filesCount) { LoadInternal(null, null, filesCount); }
|
3 修改Sample.cs代码,实现FBX动态加载
通过取消加载的位姿配置操作,可实现FBX模型初始化装配坐标配置
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
| public List<GameObject> gameObjects = new List<GameObject>(); public GameObject DynamicLoadModel(string fbxPath) { GameObject result = null; using (var assetLoader = new AssetLoader()) { try { var assetLoaderOptions = AssetLoaderOptions.CreateInstance(); assetLoaderOptions.AutoPlayAnimations = true; assetLoaderOptions.UseOriginalPositionRotationAndScale = true; var loadedGameObject = assetLoader.LoadFromFile(fbxPath, assetLoaderOptions); result = loadedGameObject as GameObject; } catch (Exception e) { Debug.LogError(e.ToString()); } } return result; }
public void LoadFBXClick() { string basePath = @"C:\Users\Administrator\Documents\SFB\Resource\ABB\base.FBX"; string fbxPath1 = @"C:\Users\Administrator\Documents\SFB\Resource\ABB\J1.FBX"; string fbxPath2 = @"C:\Users\Administrator\Documents\SFB\Resource\ABB\J2.FBX"; string fbxPath3 = @"C:\Users\Administrator\Documents\SFB\Resource\ABB\J3.FBX"; string fbxPath4 = @"C:\Users\Administrator\Documents\SFB\Resource\ABB\J4.FBX"; string fbxPath5 = @"C:\Users\Administrator\Documents\SFB\Resource\ABB\J5.FBX"; string fbxPath6 = @"C:\Users\Administrator\Documents\SFB\Resource\ABB\J6.FBX";
gameObjects.Add(this.DynamicLoadModel(basePath)); gameObjects.Add(this.DynamicLoadModel(fbxPath1)); gameObjects.Add(this.DynamicLoadModel(fbxPath2)); gameObjects.Add(this.DynamicLoadModel(fbxPath3)); gameObjects.Add(this.DynamicLoadModel(fbxPath4)); gameObjects.Add(this.DynamicLoadModel(fbxPath5)); gameObjects.Add(this.DynamicLoadModel(fbxPath6)); }
|
效果
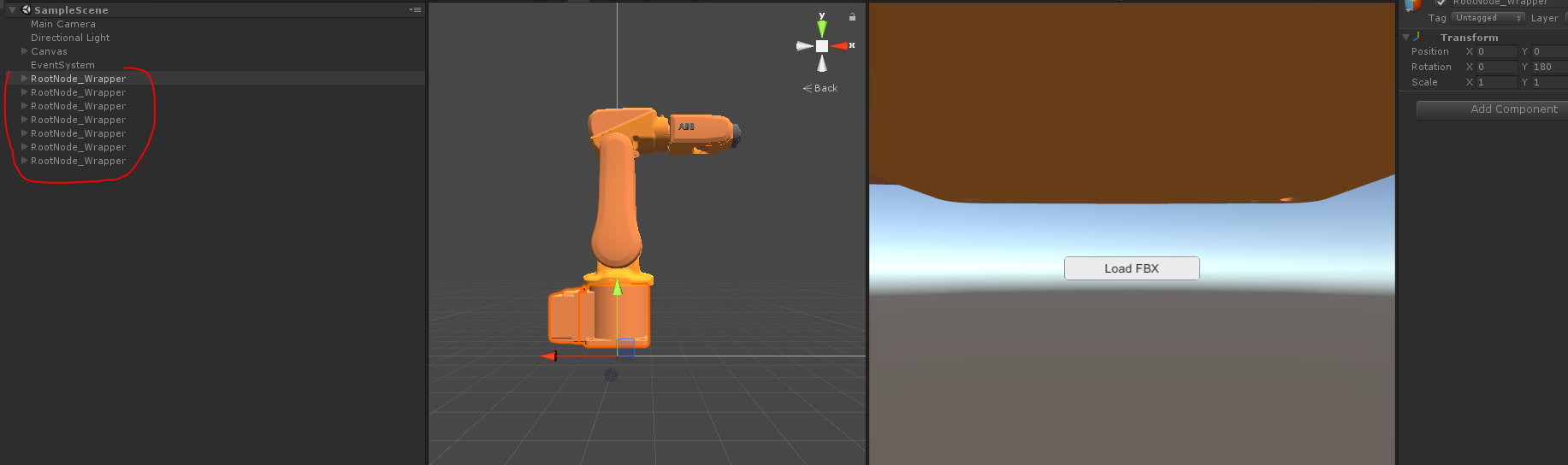